Coin Recognition using MATLAB
Objective:
The main purpose of this project is to apply edge detection techniques to develop a program which should recognize coins in an image.
For this we have used
***pre-requisite:MATLAB installed in local machine***
Image processing::
It is a method to perform some operations on an image, in order to get an enhanced image or to extract some useful information from it.
Need for coin detection-
There is a basic need to automate the counting and sorting of the coins. For this, machines need to recognize the coins very fast and accurately as further processing depends on this recognition.
The main purpose of this project is to apply computer vision techniques to develop a program which should recognize coins in an image, and enumerate their value. That is to have a computer read the image.
We have used Hugh Transform for coin detection.
[sourcecode language="MATLAB"]
clear all
close all
clc
% read image, convert into gray scale
imdata = imread('test1.jpg');
% coin is the gray image
coin = 0.2989*(imdata(:,:,1))+0.5870*(imdata(:,:,2))+0.1140*(imdata(:,:,3));
figure();
imshow(coin,'InitialMagnification','fit');
title('the grayscale image');
% edge detection
imbin = edge(coin,'canny');
imshow(imbin,'InitialMagnification','fit');
title('the edge image');
% Hough Transform
% locate the coins on RGB image
rmin=20;rmax=80; %depend on the size of coin, use possible wide range of radius
p=0.25; % quarter circle
houghcircle = HoughCoin(imbin,rmin,rmax,p);
figure();
imshow(imdata,'InitialMagnification','fit');
title('circles detected with HT');
hold on;
viscircles([houghcircle(:,1) houghcircle(:,2)], houghcircle(:,3), 'EdgeColor', 'red', 'LineWidth',4);
hold off;
[/sourcecode]
Hough Transform
[sourcecode language="MATLAB"]
function houghcircle = HoughCoin(imbin,rmin,rmax,p)
if nargin==3
p = 0.25; % accumulation to a quarter circle included
elseif nargin < 3
error('you should have more input arguements');
return;
end
% initial setting
[y0,x0] = find(imbin); % the edge pixel number
[sy,sx] = size(imbin);
totalpix = length(x0);
d = 2*rmax;
accumulator = zeros(size(imbin,1)+d,size(imbin,2)+d,rmax-rmin+1); % enlarge search area to rmax for each edge plus origin
% choose the potential circle center according to the white edge pixel
% circles location, it will not exceed the maximum radius position
% the largest dis in dectection area of each center
[m,n]=meshgrid(0:d,0:d); % only detect the square area of any points of the circle
rx = sqrt((m-rmax).^2+(n-rmax).^2); % the distance of the square is 2*rmax
R = round(rx);
R(R
[ry,rx,r_poten]= find(R);
for cnt= 1:totalpix
ind = sub2ind(size(accumulator),ry+y0(cnt)-1,rx+x0(cnt)-1,r_poten-rmin+1);
accumulator(ind) = accumulator(ind)+1;
end
% find the local maximum value of accumulator
% get rid of count less than a quarter of circle
houghcircle = []; % create a null matrix to add each filtered circle
c = 2*pi;
for radius = rmin:rmax
rlayer = accumulator(:,:,radius-rmin+1);% rlayer is the counter divided by radius
l = c*radius;
rlayer(rlayer/l
[ln,lm,num]= find(rlayer);
ratio= num/l; % ratio higher means the the possibility of center higher
houghcircle = [houghcircle;[lm-rmax, ln-rmax, radius*ones(length(lm),1),ratio]];
end
dist = 0.5*(rmin+rmax); % set as the mean value of radius
% Delete potential similar circles
houghcircle = sortrows(houghcircle,-4); % Descending sort according to possibility
for i=1:size(houghcircle,1)-1
j = i+1;
while j<= size(houghcircle,1)
if sum(abs(houghcircle(i,1:2)-houghcircle(j,1:2)))/2 <= dist
houghcircle(j,:) = [];
else
j = j+1;
end
end
end
[/sourcecode]
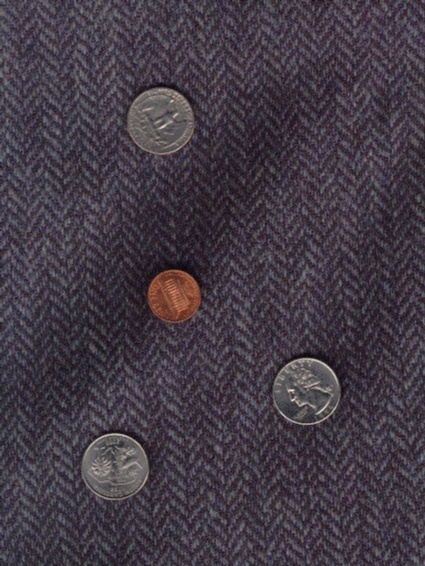
Original Image
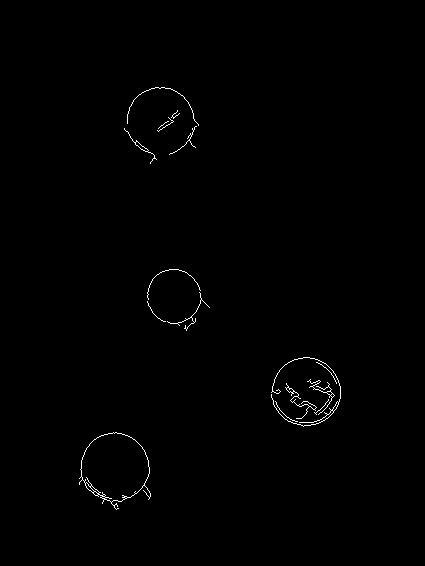
Edge Detection using Canny Filter
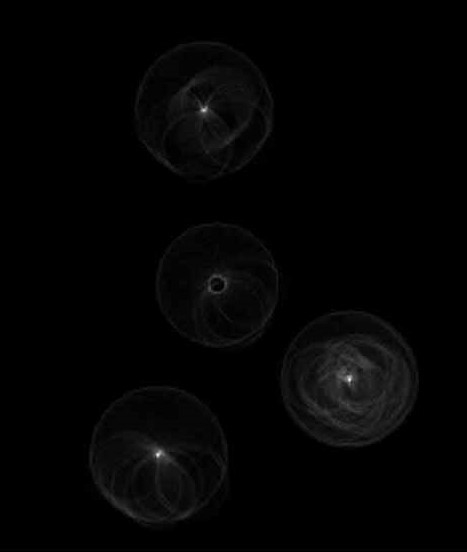
Finding Circle using Hough Transform
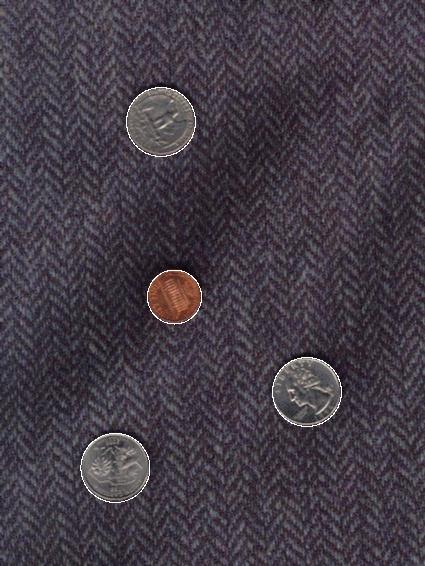
Recognised Coins
The following steps are taken in the proposed coin recognition system:
Step 1: Develop RGB code for loading database of coin image in MATLAB.
Step 2: Convert this RGB image to grayscale Image using MATLAB.
Step 3: Applied Image Thresholding on Gray Image in MATLAB.
Step 4: Crop the coin image automatically in MATLAB.
Step 5: Detection of the Edge of Image in MATLAB.
Step 6: Recognize the coin using its centroid and radius.
ratio= num/l; % ratio higher means the the possibility of center higher
houghcircle = [houghcircle;[lm-rmax, ln-rmax, radius*ones(length(lm),1),ratio]];
end
dist = 0.5*(rmin+rmax); % set as the mean value of radius
% Delete potential similar circles
houghcircle = sortrows(houghcircle,-4); % Descending sort according to possibility
for i=1:size(houghcircle,1)-1
j = i+1;
while j<= size(houghcircle,1)
if sum(abs(houghcircle(i,1:2)-houghcircle(j,1:2)))/2 <= dist
houghcircle(j,:) = [];
else
j = j+1;
end
end
end
[/sourcecode]
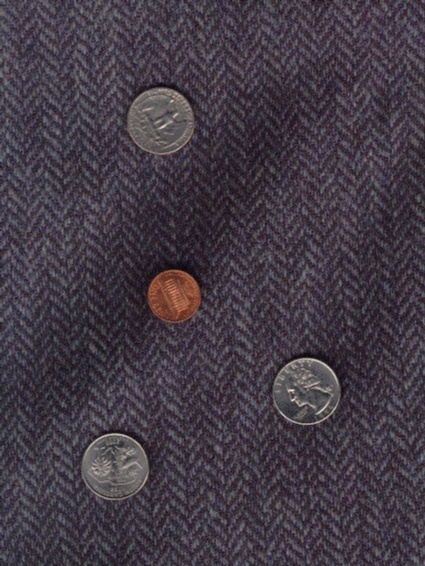
Original Image
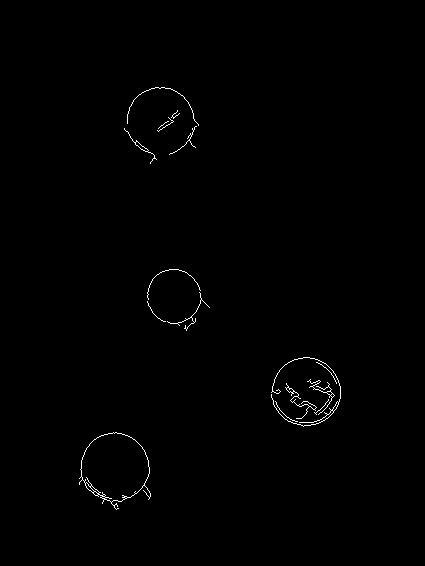
Edge Detection using Canny Filter
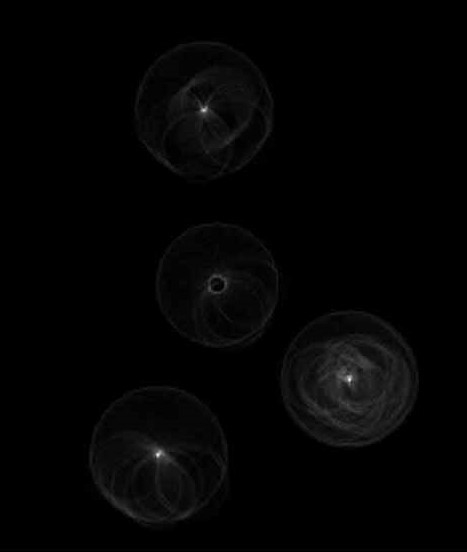
Finding Circle using Hough Transform
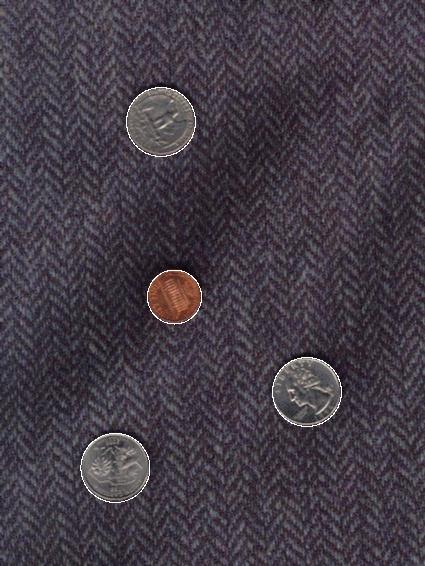
Recognised Coins
The following steps are taken in the proposed coin recognition system:
Conclusion
The proposed system can be used in coin-operated payphones, vending machines, weighing machines and in many other machines that are based on coin recognition.
The problem arises if the coin image is captured from a distance and the image tends to be small. Besides that, some of the coins are overlapped. These restrictions make the detection process difficult.
Comments
Post a Comment